Customize Splash Screen in iOS & Android with Capacitor
First impressions matter, and a clean splash screen makes your app feel professional right from the start.
With NextNative and Capacitor, adding a native and animated splash screen is super easy. Letβs walk through it.
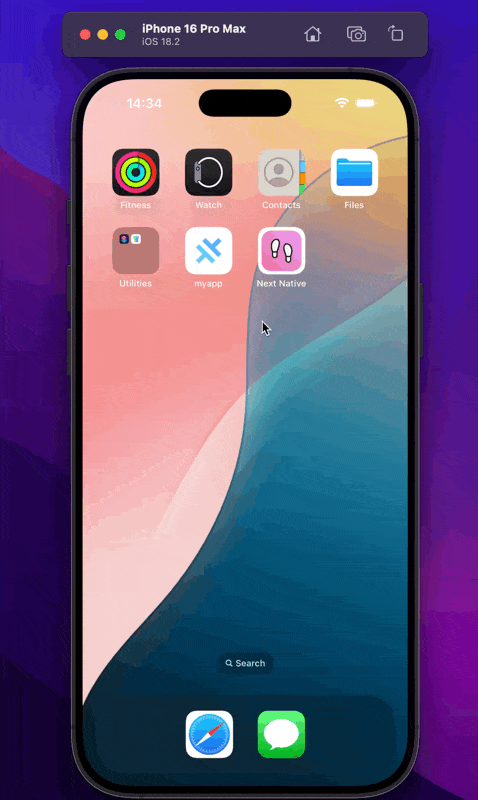
The splash screen is the first thing users see when launching your app. NextNative provides both a native splash screen (using Capacitor) and a custom in-app splash screen for a smooth transition.
Native Splash Screen
The native splash screen is managed by Capacitor and appears instantly when the app launches. You can customize it in the assets/
folder and generate (opens in a new tab) platform-specific assets using the Capacitor CLI.
In-App Splash Screen
The in-app splash screen takes over after the native splash screen and provides a smoother transition to your app:
// app/(mobile)/components/SplashScreen.tsx
import React from "react";
import { motion } from "framer-motion";
const SplashScreen = () => {
return (
<motion.div
className="fixed inset-0 bg-gradient-to-br from-blue-500 via-blue-600 to-blue-700 z-50 overflow-hidden"
initial={{ opacity: 1 }}
animate={{ opacity: 0 }}
transition={{ duration: 0.5, delay: 1.5 }}
style={{ pointerEvents: "none" }}
>
<DefaultLogo />
</motion.div>
);
};
export default SplashScreen;
This component uses Framer Motion for smooth animations. The DefaultLogo
is a simple SVG animation, but you can replace it with your own logo.
Managing the Splash Screen
NextNative provides a custom hook to manage the splash screen:
// app/(mobile)/hooks/useSplashScreen.tsx
import { useState, useEffect } from "react";
import { SplashScreen as SplashScreenPlugin } from "@capacitor/splash-screen";
export const useSplashScreen = () => {
const [showSplash, setShowSplash] = useState(true);
useEffect(() => {
const hideSplash = async () => {
await SplashScreenPlugin.hide();
setTimeout(() => {
setShowSplash(false);
}, 2000);
};
hideSplash();
}, []);
return { showSplash };
};
This hook:
- Handles the native Capacitor splash screen
- Controls the visibility of the in-app splash screen
- Returns a
showSplash
boolean to conditionally render the splash screen
Using the Splash Screen in Your App
To use the splash screen in your app, import the hook and component in your router:
// app/(mobile)/router.tsx
import { useSplashScreen } from "@/app/(mobile)/hooks/useSplashScreen";
import SplashScreen from "@/app/(mobile)/components/SplashScreen";
const AppRouter = () => {
const { showSplash } = useSplashScreen();
return (
<IonApp>
{showSplash && <SplashScreen />}
{/* Rest of your app */}
</IonApp>
);
};